✨ 배열(array)의 값(element)을 하나씩 받아서 내가 작성한 function을 실행해주는 helper method
💻Example Code
const arr = [ 1, 2, 3 ];
const received = arr.forEach( num => console.log(num) );
console.log( arr );
console.log( received );
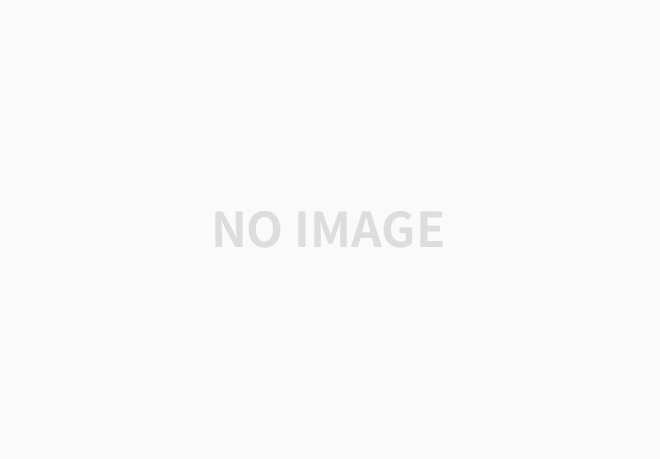
forEach는 제공 받은 function을 Array의 각 element마다 실행해주기만 한다.
function의 결과를 return 해주지 않는다. forEach의 return 값은 undefined다.
따라서 위의 결과처럼, arr를 한 번씩 실행해주고 있는 것을 볼 수 있으며, 기존 arr값이 변하지 않는 것을 볼 수 있다.
또한, return값이 있는지 확인하기 위해 선언했던 received를 출력하면 undefined가 출력되는 것을 볼 수 있다.
🎃실전 예제
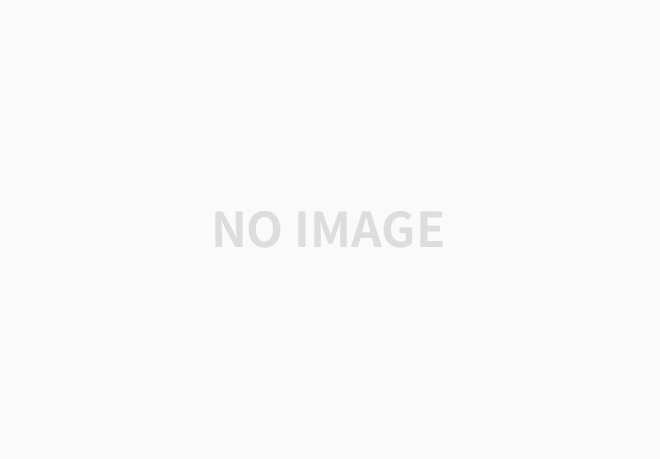
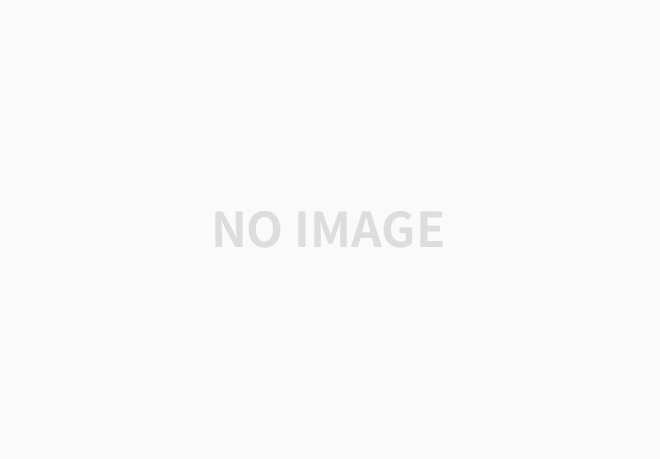
위의 간단한 예제처럼 사용할 수 있다. objArr는 직접 작성해줬지만, 어딘가에서 받아올 data라 생각하면 된다.
😋 개발을 진행할 때, 많은 양의 데이터를 받아와서 하나씩 실행해줄 때 매우 유용하다.
매우 자주 쓰이는 helper method이므로 필수적으로 알고 있어야 한다.
👉 자세한 내용은 https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
Array.prototype.forEach()
The forEach() method executes a provided function once for each array element.
developer.mozilla.org
'JavaScript > Built-in Method etc.' 카테고리의 다른 글
Math.abs() (0) | 2020.01.28 |
---|---|
Math.PI (0) | 2020.01.28 |
Math.pow() (0) | 2020.01.22 |
Math.random() (0) | 2020.01.18 |
Math.ceil() (0) | 2020.01.18 |