728x90
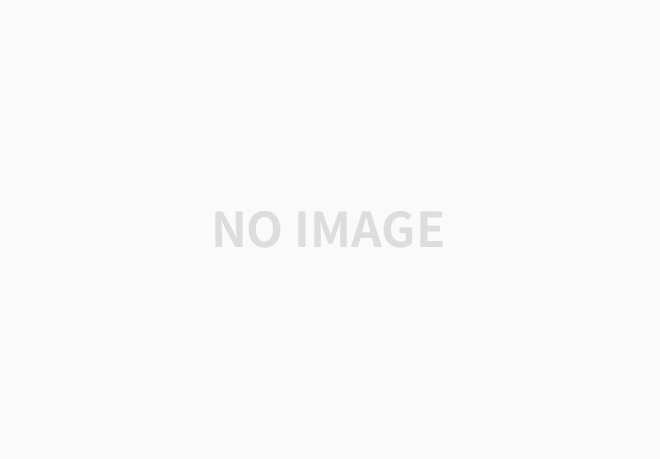
Code
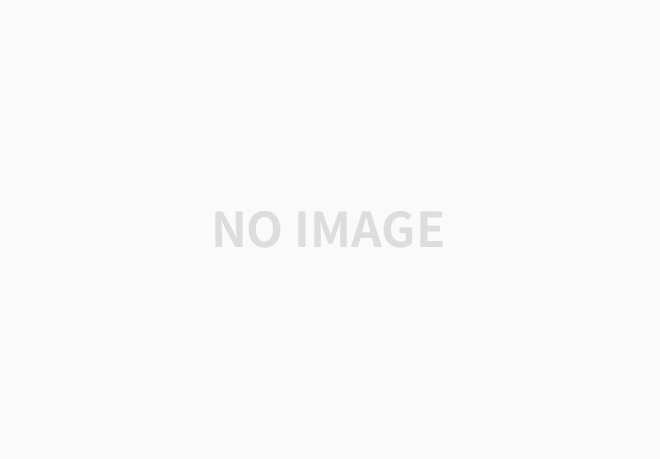
😢 역시 쉬운 문제라 내 방식대로 금방 풀었지만, 백준 형식에 맞지 않는 것인지 계속 '틀렸습니다'가 출력되었다.
이렇게 저렇게 한 10번은 바꿔보았지만, 틀린 이유를 찾지 못했다. 생각날 때마다 시도해봐야겠다. (위의 코드는 정답 코드)
😊 이번 문제에서 Function.prototype.apply()와 Number.prototype.toFixed()를 학습할 수 있었다.
✔ Function.prototype.apply() 관련
최대값을 구하기 위해서 주로 3가지 방법을 사용했었다.
1. 적은 수의 값들에서 Max를 구할 때는 'Math.max(values...)
2. classic for loop을 이용하여 하나씩 비교하여 최종 Max값을 구하는 방법
3. 백준에서는 적용되지 않지만, Math.max(...values)처럼 ES2015 'Spread'사용하여 구하는 방법
하지만, 위의 13번째 code인 Math.max.apply(null, grades) 를 이용하면 보다 쉽게 max를 구할 수 있다.
간단히 설명하자면, 두 번째 arguments에 array(or an array-like object)를 넣어주면 된다.
✔ Number.prototype.toFixed()
위의 helper method를 이용해 소수점 아래의 수를 표시할 수 있다. (e.g., toFixed(2)는 소수점 아래 2번째까지 출력)
Full Code
// 3rd Solution (submitted) |
// For Submit |
// const input = require('fs') |
// .readFileSync('/dev/stdin') |
// .toString() |
// .split('\n'); |
// For local Test |
const input = ['3', '10 20 30']; |
const N = parseInt(input[0]); |
const grades = input[1].split(' '); |
const max = Math.max.apply(null, grades); |
let sumNewGrades = 0; |
for (var i = 0; i < grades.length; i++) { |
sumNewGrades += (grades[i] / max) * 100; |
} |
console.log(sumNewGrades / N); |
// 2nd Solution (Wrong only in baekjoon) |
// For local test |
// const input = ['3', '10 20 30']; |
// const N = parseInt(input[0]); |
// const grades = input[1].split(' ').map(num => { |
// return (num = parseInt(num)); |
// }); |
// const newGrades = []; |
// let max = 0; |
// let sumNewGrades = 0; |
// let result = 0; |
// for (let i = 1; i < N; i++) { |
// if (grades[i] > max) { |
// max = grades[i]; |
// } |
// } |
// for (let grade of grades) { |
// newGrades.push((grade / max) * 100); |
// } |
// for (let grade of newGrades) { |
// sumNewGrades += grade; |
// } |
// result = sumNewGrades / newGrades.length; |
// console.log(result.toFixed(2)); |
// 1st Solution (Spread - ES2015) (Wrong only in baekjoon) |
// For local test |
// const input = ['3', '40 80 60']; |
// const N = parseInt(input[0]); |
// const grades = input[1].split(' ').map(num => (num = parseInt(num))); |
// const max = Math.max(...grades); |
// const newGrades = []; |
// let result = 0; |
// for (let grade of grades) { |
// newGrades.push((grade / max) * 100); |
// } |
// for (let grade of newGrades) { |
// result += grade; |
// } |
// console.log(result / grades.length); |
'Algorithm > JavaScript(Node.js)' 카테고리의 다른 글
Reverse Int(숫자 거꾸로 바꾸기) Node.js(JavaScript) (0) | 2019.12.24 |
---|---|
Reverse String(문자열 거꾸로 바꾸기) Node.js(JavaScript) (0) | 2019.12.23 |
백준 3052번: 나머지(The rest) Node.js(JavaScript) (0) | 2019.12.22 |
백준 2577번: 숫자의 개수(The number of numbers) Node.js(JavaScript) (0) | 2019.12.20 |
백준 2920번: 음계(scale) Node.js(JavaScript) (0) | 2019.12.20 |