728x90
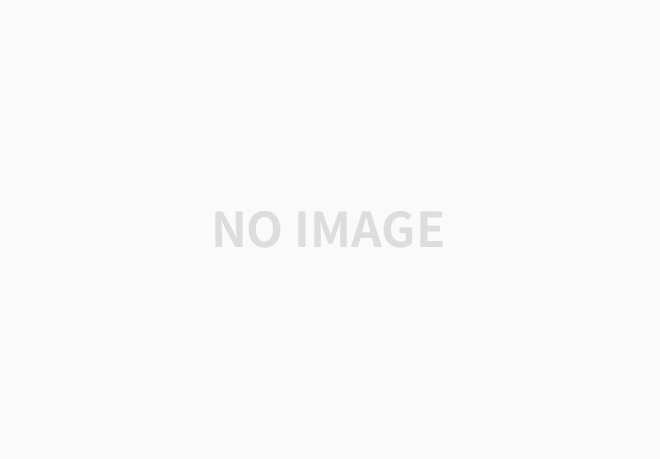
😢 정렬 문제를 많이 접해봤다면 금방 풀 수 있는 문제
😊
Solution 1)
입력 받은 단어 중 가장 큰 단어 길이(max)와 가장 작은 단어 길이(min)를 뽑아오고,
외부 for loop은 단어의 길이만큼, 내부 for loop은 입력 받은 단어 개수만큼 반복한다.
내부 for loop에서 같은 길이의 단어를 배열에 push하고, 배열 길이가 1보다 크다면 해당 배열을 정렬한 후 최종 배열에 push해준다.
이 방법으로 간단하게 해결했다.
Solution 2)
다른 풀이를 참고하던 중에, 기존에는 몰랐던 localeCompare() built-in function과 Set objet를 발견했다.
sort()로 단어의 길이만큼 정렬하고, 같은 길이의 단어가 있다면 localeCompare()를 이용해 정렬이 가능했다.
그렇게 정렬된 배열을 new Set()에 넣어주면 unique한 value를 받아볼 수 있다.
속도도 훨씬 빠르므로 Solution 2를 추천한다.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Sort words | |
// 1st Solution | |
// For submit | |
// const fs = require('fs'); | |
// const input = fs.readFileSync('/dev/stdin').toString().trim().split('\n'); | |
// For local test | |
const input = [ | |
'13', | |
'but', | |
'i', | |
'wont', | |
'hesitate', | |
'no', | |
'more', | |
'no', | |
'more', | |
'it', | |
'cannot', | |
'wait', | |
'im', | |
'yours' | |
]; | |
const N = input.shift(); | |
const sorted = []; | |
const lengthArr = input.map(str => str.length); | |
const max = Math.max.apply(null, lengthArr); | |
const min = Math.min.apply(null, lengthArr); | |
for (let i = min; i <= max; i++) { | |
const group = []; | |
for (let j = 0; j < N; j++) { | |
if (input[j].length === i) { | |
if (group.indexOf(input[j]) === -1) group.push(input[j]); | |
} | |
} | |
if (group.length > 1) { | |
sorted.push(...group.sort()); | |
} else { | |
sorted.push(...group); | |
} | |
} | |
console.log(sorted.join('\n')); | |
// 2nd Solution | |
// For submit | |
// const fs = require('fs'); | |
// const input = fs.readFileSync('/dev/stdin').toString().trim().split('\n'); | |
// For local test | |
const input = [ | |
'13', | |
'but', | |
'i', | |
'wont', | |
'hesitate', | |
'no', | |
'more', | |
'no', | |
'more', | |
'it', | |
'cannot', | |
'wait', | |
'im', | |
'yours' | |
]; | |
const N = input.shift(); | |
const sorted = input.sort((a, b) => a.length - b.length || a.localeCompare(b)); | |
const uniqueValues = new Set(sorted); | |
console.log(Array.from(uniqueValues).join('\n')); |
'Algorithm > JavaScript(Node.js)' 카테고리의 다른 글
백준 15649번: N과 M (1) Node.js(JavaScript) (0) | 2020.02.24 |
---|---|
백준 10814: 나이순 정렬 Node.js(JavaScript) (0) | 2020.02.22 |
백준 2751번: 수 정렬하기 2 Node.js(JavaScript) (0) | 2020.02.19 |
백준 11651번: 좌표 정렬하기 2 Node.js(JavaScript) (0) | 2020.02.18 |
백준 11650번: 좌표 정렬하기 Node.js(JavaScript) (0) | 2020.02.18 |